Showing county lines with embedded Google Maps
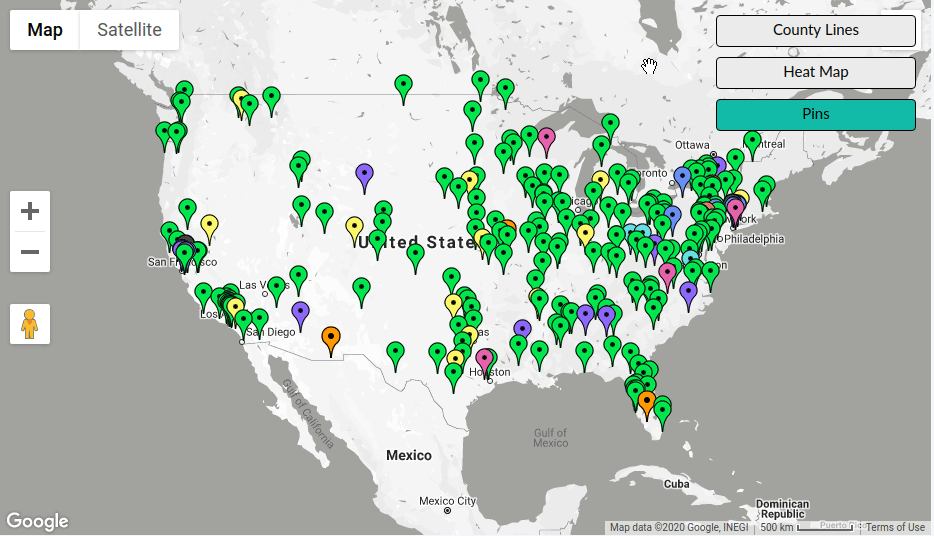
Location is important in foster care - to improve outcomes for children and youth in care it can be beneficial to place them close to their community of origin (in some but not all cases). Because foster care and other child welfare services are often administered at a county level, knowing county boundaries is also very useful.
To help caseworkers in understanding where children are placed, where available homes are, and more, Binti provides an embedded map functionality with these data points.
On the technical side, Binti had been using Fusion Tables for displaying county line boundaries, but Fusion Tables is now deprecated and no longer functioning.
Thus we needed to find an alternative and Google Maps' KML layers turned out to work well for us.
Setting up a KML Layer in Google Maps
Keyhole Markup Language or KML is a file format that describes geographic data, including regions such as counties, states, and other administrative boundaries (but also much, much more!). Google Maps is able to use KML files to display those boundaries on its embedded maps.
To take advantage of this functionality, first we need to find a KML file with the US counties. Fortunately a quick search turned up files at the US Census Bureau and NOAA. We decided to go for the latter because it was already in KMZ (compressed KML format), but either should work.
If you decide to use a large KML file, like the one from the Census Bureau, you may want to compress it because Google doesn't support KML files bigger than 5MB. A KMZ file is simply a zip file with a single KML file in the archive and the extension .kmz, which can bring down your file size into Google's acceptable range.
Next you'll need to find a place to host your KMZ or KML file publicly so that Google can find it. Some options include your webserver or an S3 bucket set up as a static website.
Finally we can use Google Maps' embedding API to point it at the publicly hosted KMZ/KML file, which it then downloads and displays over your map.
const map = new google.maps.Map(document.getElementById("map"));
const countyLayer =
new google.maps.KmlLayer({
url: "<public url of your kmz file>",
suppressInfoWindows: true,
preserveViewport: true,
map: map
});
Note that the first time you load the layer, it may take a moment as Google downloads, parses, and displays the data from your KMZ/KML file.
If you'd like to toggle the layer on and off, you can use the setMap
function on the layer like so:
// in an event handler somewhere...
if (showCounties) {
countyLayer.setMap(map);
} else {
countyLayer.setMap(null);
}
We haven't found this technique being used elsewhere, so we hope you found this post helpful!